Table of Contents
In journey of web development have you ever encountered the frustrating “Page Expired” OR 419 error in Laravel application? This error occurs when something went wrong with the CSRF token.
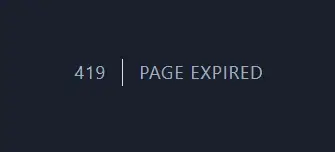
What is the Laravel 419 Error?
Generally 419 Error is occur during submit the form or make APIs in web route. Page Expired means now your session was ended or you didn’t provided CSRF token in request. Its very helpful in security of application.
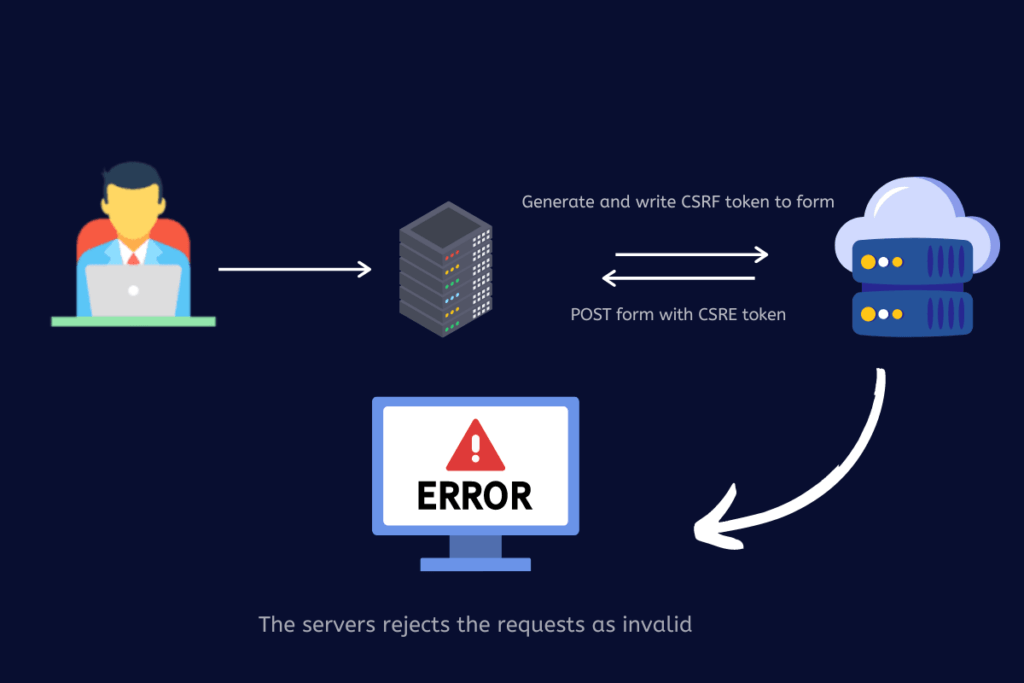
Importance of Addressing the Error
If you ignore this error, then you will lose many things. Usually, users and developers hate this error, and search engines also dislike it. If you’re thinking of removing CSRF protection, then it’s a huge mistake because removing CSRF is an invitation to spammers and unauthorized users to slow down or break your website. Without it, anybody can submit a form outside of your application, like from Postman, or maybe they write a script to create spam accounts.
What is CSRF?
CSRF (Cross-Site Request Forgery) is a security technique that prevents malicious users from submitting forms or making changes on your website without your permission. Laravel automatically adds a hidden field to forms called the CSRF token. This token is unique and ensures that the request is coming from your legitimate application.
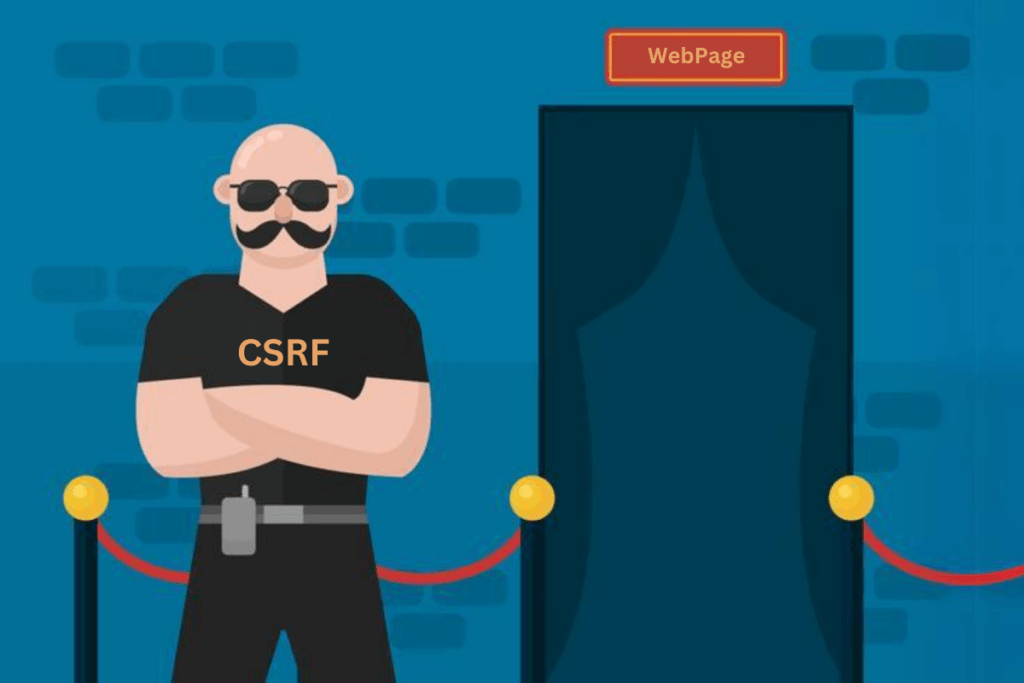
Steps to Solve the Laravel 419 Error
Refresh The Page
Refreshing page can solve error because your csrf token is valid till your session is active but if once your session was destroyed your csrf token will be invalid so to generate new csrf token you need to refresh page. this action will create new session and new csrf token so you can submit form
if your session time is very short then you can show timer to user so they can aware of this and using javascript you can refresh page automatically when session time over
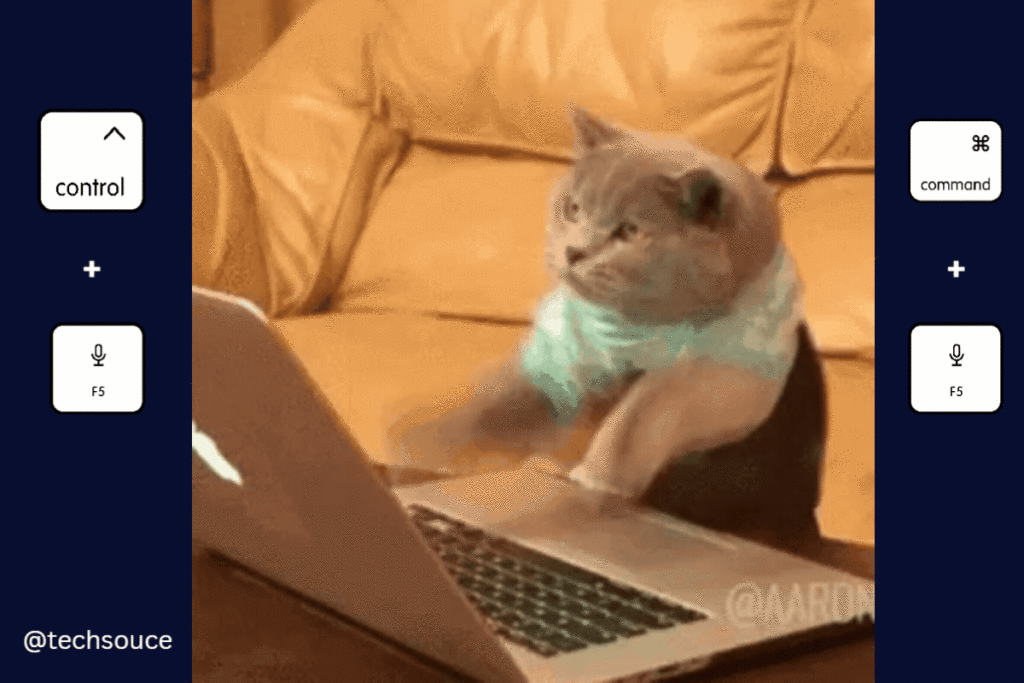
Verify the CSRF Token
Before moving forward to development process you must be aware that there is no any missing CSRF token in form or web based api request inside the code. without this line you will not able to submit form and will got 419 Error.
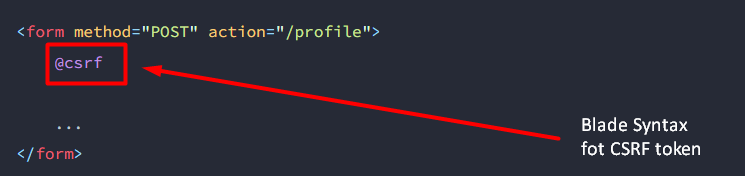
This line will add automatically hidden input field for csrf token as show below
<input name="_token" type="hidden" value="6JHXV0lIT9jdGCgVJ5tVaNLUYvUEpzD1PGx1etIr">
Session Config
After adding @csrf
in your form and still getting Laravel 419 error then you need to verify your session configuration at config/session.php
file. check all config are as below example :
'same_site' => 'lax',
'http_only' => true
check that same_site
value is 'lax'
and http_only
value is true
. These setting are required function csrf token handling and prevent to Laravel 419 Error.
Related Article: Laravel CRUD Step By Step
Verify Time & Timezone of Server
Session life is depended on time and csrf token depended on session so it’s directly relate to our Laravel 419 Error so for checking time and timezone is important go to config/app.php
file and lookup for timezone
option for Example
'timezone' => 'UTC'
make sure your server time and your timezone are synced to prevent time-related issue to that may generate Laravel 419 Error
Turn off CSRF Protection (Danger)
if you tried all above solution and still getting same error then you should go for turning off CSRF validation in your Laravel application. Commonly this is not recommended for maintain security of application. add exception pages in VerifyCsrfToken.php
to disable csrf validation into your project
app/Http/Middleware/VerifyCsrfToken.php
namespace App\Http\Middleware;
use Illuminate\Foundation\Http\Middleware\VerifyCsrfToken as Middleware;
class VerifyCsrfToken extends Middleware
{
/**
* The URIs that should be excluded from CSRF verification.
*
* @var array<int, string>
*/
protected $except = [
'/some-page',
'/some-other-page',
];
}
Remember:
- The CSRF token is sensitive and should be protected from unauthorized access.
- Always use HTTPS for secure transmission of sensitive data.