In JavaScript, understanding the distinction between null and undefined is crucial for writing robust and bug-free code. Both are primitive values, but they serve different purposes and contexts within the language.
Syntax Examples of Null and Undefined in JavaScript
Undefined: Represents a variable that has been declared but has not been assigned a value.
let x;
console.log(x); // Output: undefined
Null: Represents an intentional absence of any object value.
let y = null;
console.log(y); // Output: null
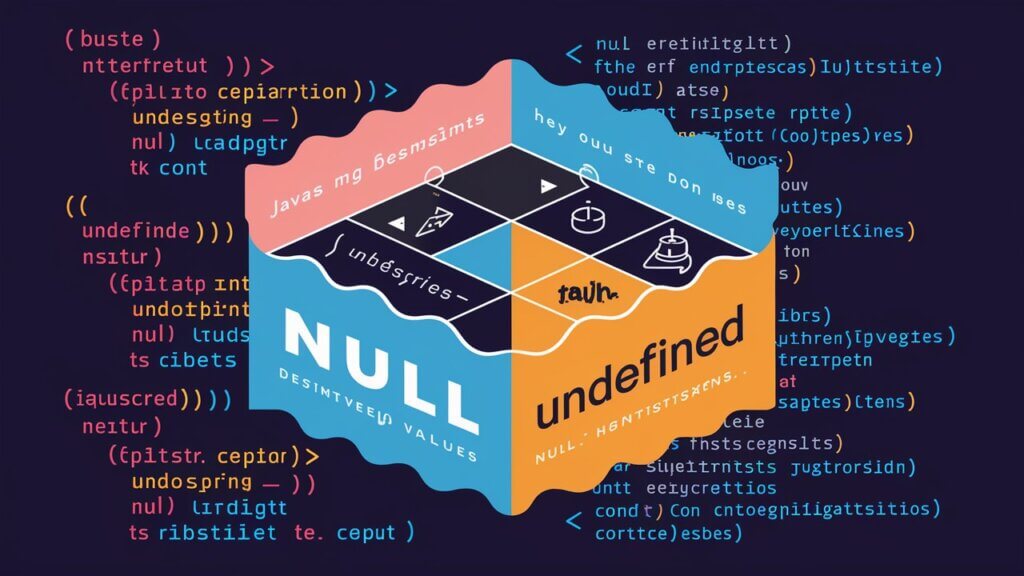
References to Null and Undefined in JavaScript
JavaScript has specific behaviors for handling both null
and undefined
values
let z;
console.log(z); // Output: undefined
let obj = {
prop: null
};
console.log(obj.prop);
undefined
is practical when the value is not existent, while null
is practical in the sense that the value is there because we know it’s not there. The user is undefined
until we fetch them, and the user becomes null
if the server returned no user.